Over the break, I decided to try my hand at some Blackberry native SDK programming since I recently got a playbook. I looked around the App World and noticed there weren’t any telnet/ssh tools available for free (that worked the way I wanted) so I thought this would be a good starter project.
However, for the look I wanted, I wanted to maximize the screen space for the console application to show as much text as possible and worked off the hello world example on the SDK webpage. Essentially, the simple “library” (note: library is used very loosely here) initializes the display, shows the keyboard and supports some simple output using putch and puts functions that I implemented. It can also show a blinking cursor and user input at a prompt. In case someone else can use the code I have made it available. Check back soon to see the source for the telnet portion of the app I made as well or look for it on the App World.
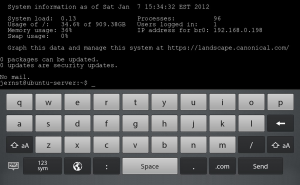
In order to use the library, you need to make use of the bbutil.c and bbutil.h files that are included in many of the examples provided by RIM. I have provided them here alongside my own code so that it is easier to follow along. I also provide two more files: glconsole.c and glconsole.h. You just need to #include “glconsole.h” in your source in order to use the library. All of the functions available to you are listed in the “glconsole.h” file as well. I will go over the important ones in this article.
First is gl_init(). This function initializes the library, the screen, font etc. This function should be called before using anything else in the library. Similarly, gl_cleanup() is used to free memory and should be called before your app is terminated.
After initialization, you may now use the puts and putch functions to display characters to the screen. gl_putch(char c) takes a single character and displays it onto the screen. It can handle newlines which are represented as ‘\n’ and tabs which are represented as ‘\t’. Backspaces by user input is handled as a ‘\b’ character. This is similar to how people on The [osdev.org](https://forum.osdev.org/) website often handle character output in their custom operating systems. I’m not sure how it relates with normal c standards, but I imagine it is similar. Eventually I hope to implement a printf() type of function, but at the moment the putch() and puts() functions serve what I am doing well enough. Note: puts() is the same as putch() but instead takes a (char *) ie) a string. The string should also be null-terminated, or it will just continue until it hits a null character. The characters are automatically scrolled when the bottom of the screen is reached.
The only other really important function is gl_render(). This function is what should be called everytime the screen needs to be refreshed. This is usually in a for loop within your main() function in your app.
It is also possible to resize the screen using the gl_resize() function. By default the screen starts at 80X29 which is the maximum size with no virtualkeyboard showing, at the font size I selected. When the keyboard shows up, it fits 80×14. In some sample code I’ll also show how keyboard input can be handled, how to blink the cursor and how to detect when the keyboard is shown or hidden.
For the rest of this guide, I’ll assume you are using the QNX Momentix IDE available from RIM’s developer website. To start, create a new project by going to File->New Blackberry Tablet OS C/C++ Project. Name it whatever you like, click next. Then choose the C language, and empty Application Project. You can now drag the gl_console.c, glconsole.h, bbutil.c and bbutil.h files into the src folder within the IDE to include them. You will probably want to copy the files and not link them when it asks. Before compiling, there are several libaries which must be linked to the project. You can do this by right clicking your project, going to properties. Then go to C/C++ General->Paths and Symbols->Libraries. Here you can add libraries. Each time you add one, be sure to check “add to all Configurations” so that you don’t need to do this again if you change from a device-debug to device-release build. The following libraries should be included:
* bps
* EGL
* GLESv1_CM
* freetype
* png
Now that all this is setup, it is possible to start you main() function and use the library. If you like you can right click on your src folder and click “new file” and create a main.c file or whatever you like to name it. While this isn’t the typical way you would include a library into a project, this way lets you edit the library source to your liking in case you want to further extend it.
The following example shows a skeleton of what is required to get the code to display some text:
```
#include <stdlib.h> /* EXIT_FAILURE */
#include <stddef.h> /* NULL */
#include <bps/bps.h> /* BPS_SUCCESS */
#include <bps/screen.h> /* screen_event_get_event() */
#include <bps/navigator.h> /* NAVIGATOR_EXIT */
#include "glconsole.h"
void handleScreenEvent(bps_event_t *event);
int main(int argc, char * argv[])
{
int exit_application = 0;
if(EXIT_SUCCESS != gl_init())
{
fprintf(stderr, "error init\n");
return 0;
}
gl_puts("Hello gl console world!\n");
for(;;)
{
bps_event_t *event = NULL;
if (bps_get_event(&event, 0) != BPS_SUCCESS)
return EXIT_FAILURE;
if (event)
{
int domain = bps_event_get_domain(event);
if (domain == screen_get_domain())
handleScreenEvent(event);
else if ((domain == navigator_get_domain()) && (NAVIGATOR_EXIT == bps_event_get_code(event)))
exit_application = 1;
if (exit_application)
break;
}
gl_render();
}
gl_cleanup();
return 0;
}
void handleScreenEvent(bps_event_t *event)
{
/* do normal event handling here, see other bb examples */
}
```
The key parts are calling gl_init() which initializes the library. Then within the main loop, the gl_render() function should be called which will actually render the text. Finally gl_cleanup() should be called before termination to properly free memory.
In the near future I plan to add some support to handle ansi encoded text (specifically for my telnet application) so that it can display colours and move the cursor around the screen using this standard.
Here are the files:
[glconsole.h](https://web.archive.org/web/20190219002308/http://www.jasonernst.com/wp-content/uploads/2012/01/glconsole.h)
[glconsole.c](https://web.archive.org/web/20190219002205/http://www.jasonernst.com/wp-content/uploads/2012/01/glconsole.c)